Using ACF-powered front end forms is quite powerful in that you can use a single ACF field group to manage a custom post type in both the WordPress admin and on the front end. This can often save a whole lot of effort as you only need to manage one ACF field group but you may find yourself in situations where you have fields that are necessary in the WordPress admin but preferably hidden from users submitted data on the front end. There are a few reasons why this might be the desired outcome:
- Less fields on the front-end mean simpler forms. This could mean better engagement.
- Some fields might only be needed internally to manage form submissions.
- Some fields might only be manageable and meaningful to site administrators.
- We don’t always want to allow users to have control over all ACF fields associated with their submission.
Fortunately, when using the Advanced Forms for ACF plugin, you have a number of ways you can mark fields for exclusion when rendering the form on the front end. In this post, I’m demonstrating three different ways to do that — one via the shortcode, one via the
advanced_form()
function, and finally a dynamic approach which you can use to implement conditional logic for the most control.
Excluding ACF form fields via the Advanced Forms shortcode
The shortcode is the most likely use case for many users as it can be added anywhere short codes are supported. Thankfully, there is a convenient shortcode arg — exclude_fields
— which can be used to specify exactly which fields not to render:
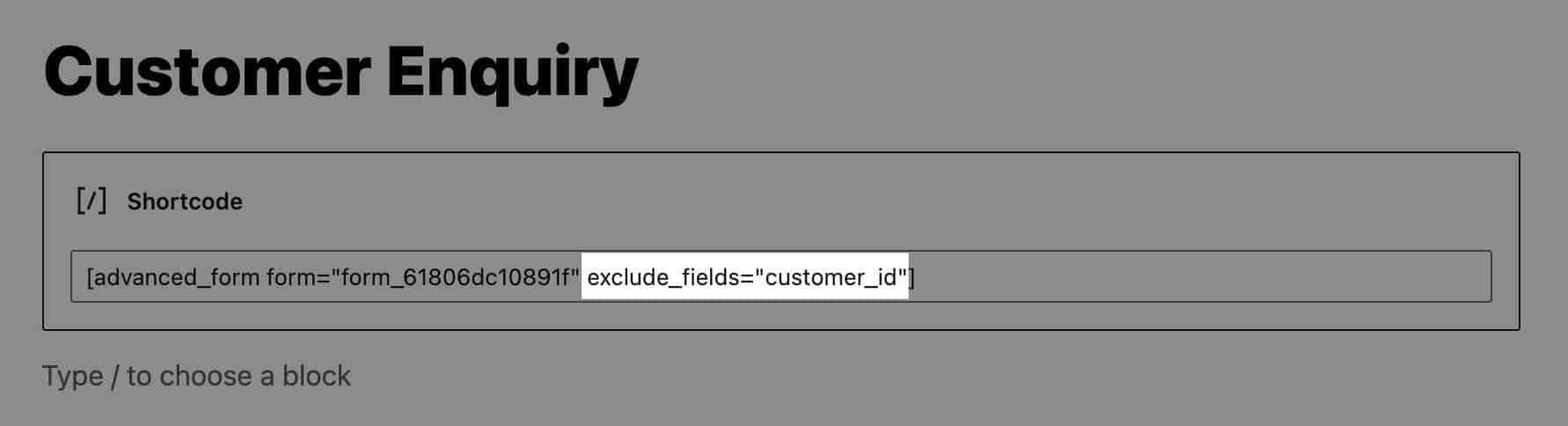
The above example is demonstrating the use of an ACF field name in the shortcode arg but be mindful that you can also pass field keys for the same result:
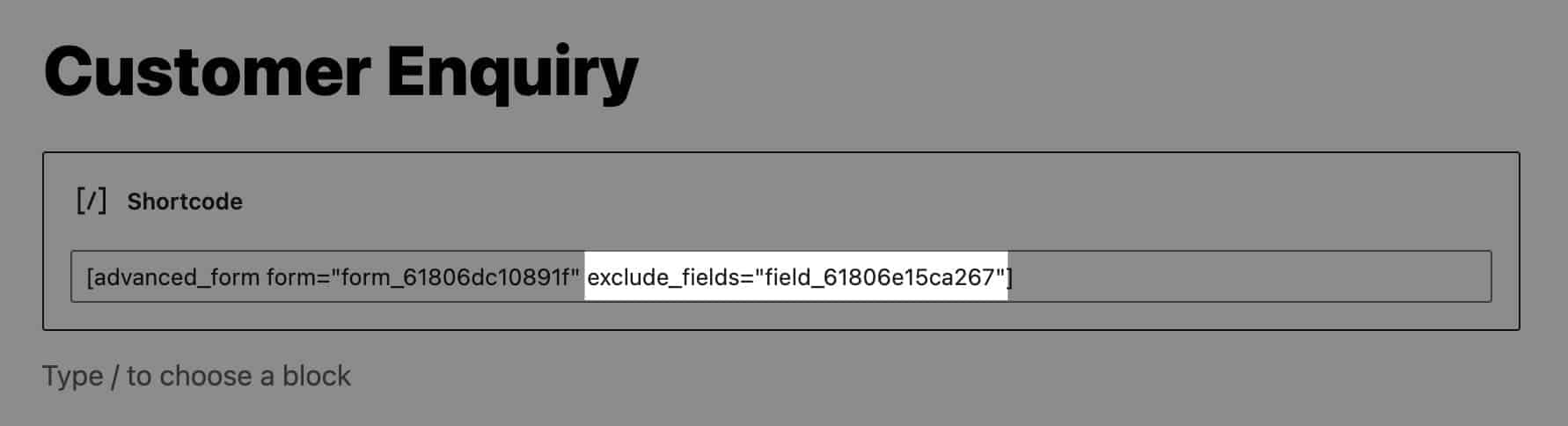
If you have multiple fields that need to be excluded from your ACF form, you can simply pass them as a comma-separated string. Note that you can use either field names or field keys and you can also use them in combination. Just be careful not to include spaces as this will be problematic.
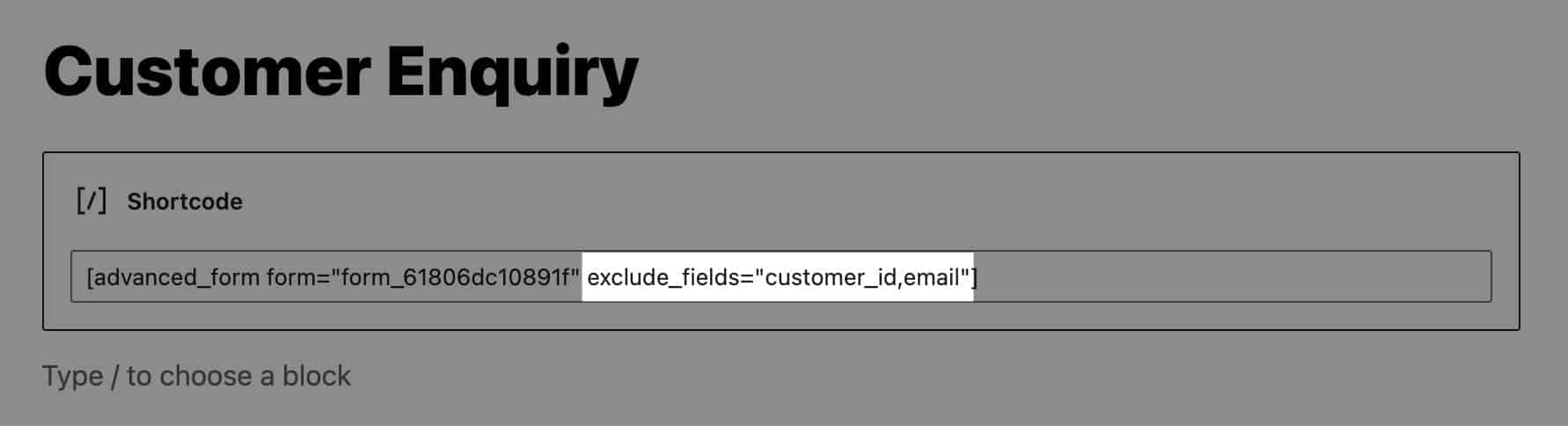
Exclude ACF form fields when using the advanced_form()
function
For those choosing to render the form using the advanced_form()
PHP function, you have the same `exclude_fields` argument available:
Just like the shortcode, the argument can support both field names and field keys:
Of course, you can pass multiple ACF field names and/or keys to exclude any number of fields:
Using a filter to dynamically control the args as the form is rendered
In addition to the configuration options, Advanced Forms Pro for ACF also provides a highly extensible set of hooks and filters that you can use to modify and customise the experience. If you need to control which fields are visible using a filter, the af/form/args filter is an excellent choice. You can use it to override the exclude_fields
argument however you need and it supports both ACF field names and field keys, as you might expect:
The real power of using a filter lies in the possibilities of dynamically changing the filter depending on external state. Perhaps a user needs a certain capability to see certain fields or maybe a URL query string parameter can be used to turn fields on and off — there are loads of possibilities here.
Here’s an example of using a custom URL query string parameter to control an ACF field’s visibility:
Wrapping up
That’s a just a simple look at one of the many extensible features available in Advanced Forms Pro. I hope this opens up new possibilities for you but if you have questions, reach out and ask.